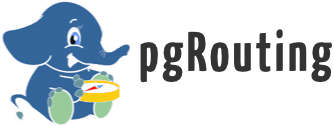 |
PGROUTING
3.2
|
Go to the documentation of this file.
26 #ifndef INCLUDE_TRSP_EDGEINFO_H_
27 #define INCLUDE_TRSP_EDGEINFO_H_
82 std::vector<size_t>
get_idx(
bool isStart)
const {
99 #endif // INCLUDE_TRSP_EDGEINFO_H_
std::vector< size_t > startConnectedEdge() const
void connect_endEdge(size_t edge_idx)
#define pgassert(expr)
Uses the standard assert syntax.
void connect_startEdge(size_t edge_idx)
std::vector< size_t > m_endConnectedEdge
An assert functionality that uses C++ throw().
std::vector< size_t > m_startConnectedEdge
double get_cost(int64_t node) const
std::vector< size_t > get_idx(bool isStart) const
std::vector< size_t > endConnectedEdge() const
int64_t startNode() const
Book keeping class for swapping orders between vehicles.