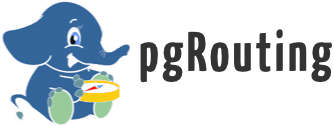 |
PGROUTING
3.2
|
Go to the documentation of this file.
35 #ifndef INCLUDE_CPP_COMMON_SIGNALHANDLER_H_
36 #define INCLUDE_CPP_COMMON_SIGNALHANDLER_H_
51 virtual const char *
what()
const throw() {
142 #define REG_SIGINT SIGINT_Handler sigint_handler; \
143 SignalHandler::instance()->registerHandler(SIGINT, &sigint_handler);
145 #define REG_SIGQUIT SIGQUIT_Handler sigquit_handler; \
146 SignalHandler::instance()->registerHandler(SIGQUIT, &sigquit_handler);
148 #define THROW_ON_SIGINT do { \
149 if ( sigint_handler.gracefulQuit() == 1 ) \
150 throw(UserQuitException("Abort on User Request!")); \
153 #endif // INCLUDE_CPP_COMMON_SIGNALHANDLER_H_
virtual void handleSignal(int signum)
static void dispatcher(int signum)
static EventHandler * signalHandlers_[NSIG]
virtual void handleSignal(int signum)=0
sig_atomic_t gracefulQuit(void)
virtual void handleSignal(int signum)
UserQuitException(const char *_str)
sig_atomic_t abortiveQuit(void)
sig_atomic_t abortive_quit_
static SignalHandler * instance_
EventHandler * registerHandler(int signum, EventHandler *eh)
static SignalHandler * instance(void)
void removeHandler(int signum)
sig_atomic_t graceful_quit_
virtual const char * what() const
const char * str
str Holds the what() string for the exception.