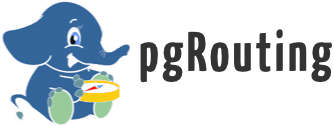 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_VEHICLE_H_
29 #define INCLUDE_VRP_VEHICLE_H_
48 class Pgr_pickDeliver;
90 typedef std::tuple< int, int, size_t, double, double >
Cost;
91 std::vector<General_vehicle_orders_t>
106 double speed()
const;
202 return m_path.back().departure_time();
205 return m_path.back().total_wait_time();
208 return m_path.back().total_travel_time();
211 return m_path.back().total_service_time();
214 return m_path.back().twvTot();
217 return m_path.back().cvTot();
293 std::deque< Vehicle_node >
path()
const;
303 std::string
tau()
const;
329 #endif // INCLUDE_VRP_VEHICLE_H_
std::deque< Vehicle_node > m_path
POS getPosHighLimit(const Vehicle_node &node) const
std::vector< General_vehicle_orders_t > get_postgres_result(int vid) const
Extend Tw_node to evaluate the vehicle at node level.
size_t size() const
return number of nodes in the truck
POS getDropPosLowLimit(const Vehicle_node &node) const
POS getPosLowLimit(const Vehicle_node &node) const
friend bool operator<(const Vehicle &lhs, const Vehicle &rhs)
std::deque< Vehicle_node > path() const
@ {
Vehicle with time windows.
double total_service_time() const
Vehicle_node end_site() const
std::deque< Vehicle_node >::difference_type difference_type
std::pair< POS, POS > drop_position_limits(const Vehicle_node node) const
void invariant() const
Invariant The path must:
friend std::ostream & operator<<(std::ostream &log, const Vehicle &v)
@ {
double total_travel_time() const
std::pair< POS, POS > position_limits(const Vehicle_node node) const
double total_wait_time() const
bool empty() const
return true when no nodes are in the truck
static Pgr_pickDeliver * problem
Pointer to problem.
Vehicle_node start_site() const
Vehicle(const Vehicle &)=default
bool cost_compare(const Cost &, const Cost &) const
void insert(POS pos, Vehicle_node node)
@ {
std::tuple< int, int, size_t, double, double > Cost
Book keeping class for swapping orders between vehicles.
void swap(POS i, POS j)
Swap two nodes in the path.
static Pgr_messages & msg()
Access to the problem's message.
void erase(const Vehicle_node &node)
Erase node.id()