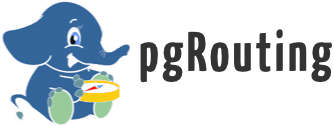 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_TRSP_GRAPHDEFINITION_H_
29 #define INCLUDE_TRSP_GRAPHDEFINITION_H_
48 typedef std::pair<int64, bool>
PIB;
49 typedef std::pair<double, PIB>
PDP;
50 typedef std::pair<double, std::vector<int64> >
PDVI;
85 typedef std::map<int64, std::vector<Rule> >
RuleTable;
121 int64 start_vertex, int64 end_vertex,
122 bool directed,
bool has_reverse_cost,
127 int64 start_vertex, int64 end_vertex,
128 bool directed,
bool has_reverse_cost,
131 std::vector<PDVI> &ruleList);
134 int64 start_edge,
double start_part,
135 int64 end_edge,
double end_part,
136 bool directed,
bool has_reverse_cost,
139 std::vector<PDVI> &ruleList);
142 bool has_reverse_cost,
bool directed);
149 std::vector<PDP>, std::greater<PDP> > &que);
154 bool bIsStartNodeSame);
181 #endif // INCLUDE_TRSP_GRAPHDEFINITION_H_
Long2LongVectorMap m_mapNodeId2Edge
std::vector< path_element_tt > m_vecPath
GraphEdgeVector m_vecEdgeVector
bool addEdge(edge_t edgeIn)
std::pair< int64, bool > PIB
LongVector m_vecEndConnedtedEdge
LongVector m_vecStartConnectedEdge
std::vector< LongVector > VectorOfLongVector
std::pair< double, std::vector< int64 > > PDVI
std::vector< GraphEdgeInfo * > GraphEdgeVector
Rule(double c, std::vector< int64 > p)
bool m_bIsLeadingRestrictedEdge
void explore(int64 cur_node, GraphEdgeInfo &cur_edge, bool isStart, LongVector &vecIndex, std::priority_queue< PDP, std::vector< PDP >, std::greater< PDP > > &que)
bool connectEdge(GraphEdgeInfo &firstEdge, GraphEdgeInfo &secondEdge, bool bIsStartNodeSame)
std::map< int64, int64 > Long2LongMap
std::pair< double, PIB > PDP
Long2LongMap m_mapEdgeId2Index
std::vector< int64 > precedencelist
bool construct_graph(edge_t *edges, size_t edge_count, bool has_reverse_cost, bool directed)
double construct_path(int64 ed_id, int64 v_pos)
VectorOfLongVector m_vecRestrictedEdge
std::map< int64, std::vector< Rule > > RuleTable
bool get_single_cost(double total_cost, path_element_tt **path, size_t *path_count)
std::map< int64, LongVector > Long2LongVectorMap
bool m_bIsGraphConstructed
int my_dijkstra3(edge_t *edges, size_t edge_count, int64 start_vertex, int64 end_vertex, bool directed, bool has_reverse_cost, path_element_tt **path, size_t *path_count, char **err_msg)
std::vector< int64 > LongVector
int my_dijkstra1(edge_t *edges, size_t edge_count, int64 start_edge, double start_part, int64 end_edge, double end_part, bool directed, bool has_reverse_cost, path_element_tt **path, size_t *path_count, char **err_msg, std::vector< PDVI > &ruleList)
double getRestrictionCost(int64 cur_node, GraphEdgeInfo &new_edge, bool isStart)
int my_dijkstra2(edge_t *edges, size_t edge_count, int64 start_vertex, int64 end_vertex, bool directed, bool has_reverse_cost, path_element_tt **path, size_t *path_count, char **err_msg, std::vector< PDVI > &ruleList)