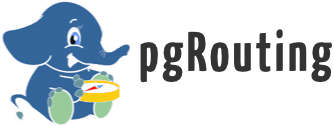 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_BOOK_KEEPING_H_
29 #define INCLUDE_VRP_BOOK_KEEPING_H_
59 <<
"\n" << d.from_truck.tau() <<
" --> "
62 << d.from_truck.orders()[d.from_order].pickup().original_id()
64 <<
"\n" << d.to_truck.tau() <<
" --> "
67 << d.to_truck.orders()[d.to_order].pickup().original_id()
69 <<
"\n" <<
"delta = " << d.estimated_delta;
93 typedef std::priority_queue<
95 std::vector<Swap_info>,
115 #endif // INCLUDE_VRP_BOOK_KEEPING_H_
void push(const Swap_info &data)
std::priority_queue< Swap_info, std::vector< Swap_info >, Compare > Swaps_queue
Swaps_queue & possible_swaps()
Vehicle_pickDeliver from_truck
Vehicle_pickDeliver to_truck
friend std::ostream & operator<<(std::ostream &log, const Swap_info &d)
bool operator()(const Swap_info &lhs, const Swap_info rhs)
friend std::ostream & operator<<(std::ostream &log, const Swap_bk &data)
Book keeping class for swapping orders between vehicles.