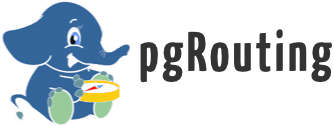 |
PGROUTING
3.2
|
Go to the documentation of this file.
33 #ifndef INCLUDE_CPP_COMMON_IDENTIFIERS_HPP_
34 #define INCLUDE_CPP_COMMON_IDENTIFIERS_HPP_
51 typedef typename std::set<T>::iterator
iterator;
69 std::generate_n(std::inserter(
m_ids,
m_ids.begin()),
71 [&i](){ return i++; });
98 bool has(
const T other)
const {
141 m_ids.insert(element);
163 std::set_intersection(
166 std::inserter(result, result.begin()));
176 *
this = *
this * other;
187 m_ids.insert(element);
213 std::inserter(result, result.begin()));
225 *
this = *
this - other;
234 m_ids.erase(element);
245 for (
auto identifier : identifiers.
m_ids) {
246 os << identifier <<
", ";
255 #endif // INCLUDE_CPP_COMMON_IDENTIFIERS_HPP_
Identifiers< T > & operator*=(const Identifiers< T > &other)
coumpound set INTERSECTION set
friend Identifiers< T > operator-(const Identifiers< T > &lhs, const Identifiers< T > &rhs)
friend Identifiers< T > operator+(const Identifiers< T > &lhs, const Identifiers< T > &rhs)
set UNION set
friend Identifiers< T > operator*(const Identifiers< T > &lhs, const Identifiers< T > &rhs)
set INTERSECTION
const_iterator begin() const
Identifiers< T > & operator+=(const Identifiers< T > &other)
compound set UNION set
std::set< T >::iterator iterator
const_iterator end() const
bool has(const T other) const
true ids() has element
friend std::ostream & operator<<(std::ostream &os, const Identifiers< T > &identifiers)
Prints the set of identifiers.
bool operator==(const Identifiers< T > &rhs) const
true when both sets are equal
Identifiers< T > & operator-=(const Identifiers< T > &other)
compound set DIFFERENCE set
std::set< T >::const_iterator const_iterator