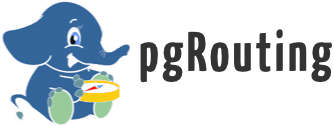 |
PGROUTING
3.2
|
Go to the documentation of this file.
27 #ifndef INCLUDE_MAX_FLOW_PGR_MINCOSTMAXFLOW_HPP_
28 #define INCLUDE_MAX_FLOW_PGR_MINCOSTMAXFLOW_HPP_
40 #include <boost/graph/successive_shortest_path_nonnegative_weights.hpp>
41 #include <boost/graph/find_flow_cost.hpp>
52 typedef Traits::vertex_descriptor
V;
53 typedef Traits::edge_descriptor
E;
54 typedef boost::graph_traits<CostFlowGraph>::vertex_iterator
V_it;
55 typedef boost::graph_traits<CostFlowGraph>::edge_iterator
E_it;
64 boost::successive_shortest_path_nonnegative_weights(
68 return boost::find_flow_cost(
graph);
74 const std::vector<pgr_costFlow_t> &edges,
75 const std::set<int64_t> &source_vertices,
76 const std::set<int64_t> &sink_vertices);
98 const std::set<int64_t> &source_vertices);
100 const std::set<int64_t> &sink_vertices);
105 const std::vector<pgr_costFlow_t> &edges);
110 template <
typename T>
113 const std::set<int64_t> &source_vertices,
114 const std::set<int64_t> &sink_vertices) {
115 std::set<int64_t> vertices(source_vertices);
116 vertices.insert(sink_vertices.begin(), sink_vertices.end());
118 for (
const auto e : edges) {
119 vertices.insert(e.source);
120 vertices.insert(e.target);
123 for (
const auto id : vertices) {
125 idToV.insert(std::pair<int64_t, V>(
id, v));
126 vToId.insert(std::pair<V, int64_t>(v,
id));
151 #endif // INCLUDE_MAX_FLOW_PGR_MINCOSTMAXFLOW_HPP_
std::map< int64_t, V > idToV
PgrCostFlowGraph()=delete
boost::adjacency_list< boost::vecS, boost::vecS, boost::directedS, boost::no_property, boost::property< boost::edge_capacity_t, double, boost::property< boost::edge_residual_capacity_t, double, boost::property< boost::edge_reverse_t, Traits::edge_descriptor, boost::property< boost::edge_weight_t, double > > > > > CostFlowGraph
ResidualCapacity residual_capacity
std::map< V, int64_t > vToId
void SetSupersource(const std::set< int64_t > &source_vertices)
boost::graph_traits< CostFlowGraph >::edge_iterator E_it
V GetBoostVertex(int64_t id) const
int64_t GetVertexId(V v) const
boost::graph_traits< CostFlowGraph >::vertex_iterator V_it
Traits::vertex_descriptor V
boost::property_map< CostFlowGraph, boost::edge_capacity_t >::type Capacity
int64_t GetMaxFlow() const
boost::property_map< CostFlowGraph, boost::edge_residual_capacity_t >::type ResidualCapacity
void SetSupersink(const std::set< int64_t > &sink_vertices)
E AddEdge(V v, V w, double wei, double cap)
void AddVertices(const T &edges, const std::set< int64_t > &source_vertices, const std::set< int64_t > &sink_vertices)
std::map< E, int64_t > eToId
boost::property_map< CostFlowGraph, boost::edge_reverse_t >::type Reversed
std::vector< pgr_flow_t > GetFlowEdges() const
Traits::edge_descriptor E
int64_t GetEdgeId(E e) const
boost::property_map< CostFlowGraph, boost::edge_weight_t >::type Weight
Book keeping class for swapping orders between vehicles.
void InsertEdges(const std::vector< pgr_costFlow_t > &edges)