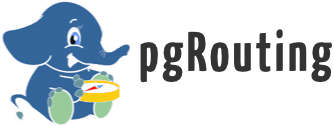 |
PGROUTING
3.2
|
Go to the documentation of this file.
26 #ifndef INCLUDE_CPP_COMMON_RULE_H_
27 #define INCLUDE_CPP_COMMON_RULE_H_
40 using iterator = std::vector<int64_t>::iterator;
46 inline double cost()
const {
78 #endif // INCLUDE_CPP_COMMON_RULE_H_
const std::vector< int64_t > precedencelist() const
std::vector< int64_t > m_precedencelist
std::vector< int64_t > m_all
std::vector< int64_t >::const_iterator constiterator
friend std::ostream & operator<<(std::ostream &log, const Rule &r)
std::vector< int64_t >::iterator iterator
constiterator begin() const
constiterator end() const
Book keeping class for swapping orders between vehicles.