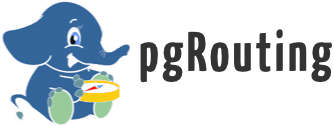 |
PGROUTING
3.2
|
Go to the documentation of this file.
26 #ifndef INCLUDE_TSP_TOUR_H_
27 #define INCLUDE_TSP_TOUR_H_
48 explicit Tour(
const std::vector<size_t> &cities_order) :
162 #endif // INCLUDE_TSP_TOUR_H_
double tourCost(const Tour &tour) const
tour evaluation
std::vector< size_t >::difference_type difference_type
std::vector< size_t > cities
void rotate(size_t c1, size_t c2, size_t c3)
void swap(size_t c1, size_t c2)
void slide(size_t place, size_t first, size_t last)
Tour(const std::vector< size_t > &cities_order)
Tour(const Tour &)=default
Book keeping class for swapping orders between vehicles.
void reverse(size_t c1, size_t c2)
friend std::ostream & operator<<(std::ostream &log, const Tour &tour)