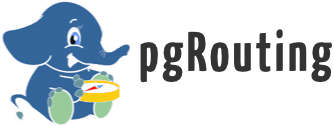 |
PGROUTING
3.2
|
Go to the documentation of this file.
53 const std::vector<pgrouting::trsp::Rule> &restrictions,
82 size_t total_restrictions,
99 std::ostringstream log;
100 std::ostringstream err;
101 std::ostringstream notice;
110 std::vector<pgrouting::trsp::Rule> ruleList;
111 for (
size_t i = 0; i < total_restrictions; ++i) {
112 ruleList.push_back(
Rule(*(restrictions + i)));
115 log <<
"\n---------------------------------------\nRestrictions data\n";
116 for (
const auto &r : ruleList) {
119 log <<
"------------------------------------------------------------\n";
123 std::vector < pgr_edge_t > edges(data_edges, data_edges + total_edges);
125 std::deque<Path> paths;
129 log <<
"Working with directed Graph\n";
146 log <<
"TODO Working with Undirected Graph\n";
167 log <<
"\nCount = " << count;
170 *return_tuples = NULL;
171 *return_tuples =
pgr_alloc(count, (*return_tuples));
175 for (
const auto &path : paths) {
176 if (path.size() > 0) {
177 path.get_pg_turn_restricted_path(
182 log <<
"the agg cost" << (*return_tuples)[0].agg_cost;
186 *return_count = count;
190 *log_msg = log.str().empty()?
193 *notice_msg = notice.str().empty()?
198 (*return_tuples) =
pgr_free(*return_tuples);
200 err << except.
what();
201 *err_msg =
pgr_msg(err.str().c_str());
202 *log_msg =
pgr_msg(log.str().c_str());
203 }
catch (std::exception &except) {
204 (*return_tuples) =
pgr_free(*return_tuples);
206 err << except.what();
207 *err_msg =
pgr_msg(err.str().c_str());
208 *log_msg =
pgr_msg(log.str().c_str());
210 (*return_tuples) =
pgr_free(*return_tuples);
212 err <<
"Caught unknown exception!";
213 *err_msg =
pgr_msg(err.str().c_str());
214 *log_msg =
pgr_msg(log.str().c_str());
T * pgr_alloc(std::size_t size, T *ptr)
allocates memory
size_t count_tuples(const std::deque< Path > &paths)
void do_pgr_turnRestrictedPath(pgr_edge_t *data_edges, size_t total_edges, Restriction_t *restrictions, size_t total_restrictions, int64_t start_vid, int64_t end_vid, size_t k, bool directed, bool heap_paths, bool stop_on_first, bool strict, General_path_element_t **return_tuples, size_t *return_count, char **log_msg, char **notice_msg, char **err_msg)
char * pgr_msg(const std::string &msg)
virtual const char * what() const
#define pgassert(expr)
Uses the standard assert syntax.
void insert_edges(const T *edges, size_t count)
Inserts count edges of type T into the graph.
std::string get_log() const
get_log
An assert functionality that uses C++ throw().
std::deque< Path > turnRestrictedPath(G &graph, const std::vector< pgrouting::trsp::Rule > &restrictions, int64_t source, int64_t target, size_t k, bool heap_paths, bool stop_on_first, bool strict)
graph::Pgr_base_graph< BG, XY_vertex, Basic_edge > G
static std::deque< Path > pgr_dijkstraTR(G &graph, const std::vector< pgrouting::trsp::Rule > &restrictions, int64_t source, int64_t target, std::string &log, size_t k, bool heap_paths, bool stop_on_first, bool strict)
Extends std::exception and is the exception that we throw if an assert fails.