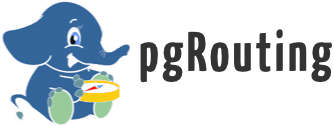 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_VEHICLE_PICKDELIVER_H_
29 #define INCLUDE_VRP_VEHICLE_PICKDELIVER_H_
44 class Initial_solution;
195 #endif // INCLUDE_VRP_VEHICLE_PICKDELIVER_H_
Identifiers< size_t > m_orders_in_vehicle
orders inserted in this vehicle
void erase(const Order &order)
const PD_Orders & orders() const
void push_back(const Order &order)
puts an order at the end of the truck
bool semiLIFO(const Order &order)
Inserts an order In semi-Lifo order.
Extend Tw_node to evaluate the vehicle at node level.
void push_front(const Order &order)
Puts an order at the end front of the truck.
Identifiers< size_t > m_feasable_orders
orders that fit in the truck
void set_compatibles(const PD_Orders &orders)
Vehicle with time windows.
bool is_order_feasable(const Order &order) const
size_t orders_size() const
Identifiers< size_t > feasable_orders() const
bool insert(const Order &order)
Inserts an order.
Identifiers< size_t > orders_in_vehicle() const
bool has_order(const Order &order) const
Vehicle_pickDeliver(size_t idx, int64_t id, const Vehicle_node &starting_site, const Vehicle_node &ending_site, double p_capacity, double p_speed, double factor)
Initials_code
Different kinds to insert an order into the vehicle.
Book keeping class for swapping orders between vehicles.
void do_while_feasable(Initials_code kind, Identifiers< size_t > &unassigned, Identifiers< size_t > &assigned)