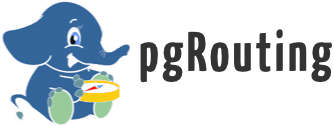 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_CPP_COMMON_DMATRIX_H_
29 #define INCLUDE_CPP_COMMON_DMATRIX_H_
46 explicit Dmatrix(
const std::vector < Matrix_cell_t > &data_costs);
47 explicit Dmatrix(
const std::map<std::pair<double, double>, int64_t> &euclidean_data);
60 void set(
size_t i,
size_t j,
double dist) {
68 bool has_id(int64_t
id)
const;
82 int64_t
get_id(
size_t idx)
const;
102 const std::vector<double>&
get_row(
size_t idx)
const {
123 void set_ids(
const std::vector<matrix_cell> &data_costs);
127 typedef std::vector < std::vector < double > >
Costs;
136 #endif // INCLUDE_CPP_COMMON_DMATRIX_H_
size_t get_index(int64_t id) const
original id -> idx
void set(size_t i, size_t j, double dist)
sets a special value for the distance(i,j)
double tourCost(const Tour &tour) const
tour evaluation
double comparable_distance(size_t i, size_t j) const
bool is_symmetric() const
bool has_no_infinity() const
bool has_id(int64_t id) const
original id -> true
std::vector< int64_t > ids
std::vector< std::vector< double > > Costs
int64_t get_id(size_t idx) const
idx -> original id
const std::vector< double > & get_row(size_t idx) const
returns a row of distances
bool obeys_triangle_inequality() const
Triangle Inequality Theorem.
void set_ids(const std::vector< matrix_cell > &data_costs)
double distance(int64_t i, int64_t j) const
std::vector< double > & operator[](size_t i)
friend std::ostream & operator<<(std::ostream &log, const Dmatrix &matrix)
double distance(size_t i, size_t j) const
Book keeping class for swapping orders between vehicles.