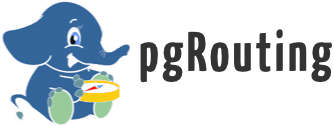 |
PGROUTING
3.2
|
Go to the documentation of this file.
27 #ifndef INCLUDE_CPP_COMMON_BASEPATH_SSEC_HPP_
28 #define INCLUDE_CPP_COMMON_BASEPATH_SSEC_HPP_
32 #include <boost/config.hpp>
33 #include <boost/graph/adjacency_list.hpp>
48 typedef std::deque< Path_t >::iterator
pthIt;
60 Path(int64_t s_id, int64_t e_id)
120 friend std::ostream&
operator<<(std::ostream &log,
const Path &p);
135 size_t &sequence)
const;
139 size_t &sequence,
int routeId)
const;
143 size_t &sequence,
int routeId)
const;
147 size_t &sequence)
const;
151 const std::deque< Path > &paths);
155 friend void equi_cost(std::deque< Path > &paths);
157 friend size_t count_tuples(
const std::deque< Path > &paths);
162 template <
typename G ,
typename V>
Path(
166 const std::vector<V> &predecessors,
167 const std::vector<double> &distances) :
170 for (
V i = 0; i < distances.size(); ++i) {
171 if (distances[i] <= distance) {
172 auto cost = distances[i] - distances[predecessors[i]];
173 auto edge_id = graph.get_edge_id(predecessors[i], i, cost);
185 const Path &original,
190 if (original.
path.empty())
return;
192 typename G::EO_i ei, ei_end;
195 for (
const auto &p : original.
path) {
196 boost::tie(ei, ei_end) = out_edges(
203 for ( ; ei != ei_end; ++ei) {
204 if (graph[*ei].
id == p.edge) {
205 auto cost = graph[*ei].cost;
224 template <
typename G ,
typename V>
Path(
228 const std::vector<V> &predecessors,
229 const std::vector<double> &distances) :
231 m_end_id(graph.graph[v_source].id) {
232 for (
V i = 0; i < distances.size(); ++i) {
233 if (distances[i] <= distance) {
234 auto cost = distances[i] - distances[predecessors[i]];
235 auto edge_id = graph.get_edge_id(predecessors[i], i, cost);
246 template <
typename G ,
typename V>
Path(
250 const std::vector<V> &predecessors,
251 const std::vector<double> &distances,
253 bool normal =
true) :
255 m_end_id(graph.graph[v_target].id) {
268 if (v_target != predecessors[v_target]) {
270 {graph.graph[v_target].id,
273 distances[v_target]});
286 const std::vector<V> &predecessors,
287 const std::vector<double> &distances,
290 if (v_target == predecessors[v_target]) {
297 auto target = v_target;
303 {graph.graph[target].id, -1,
304 0, distances[target]});
309 while (target != v_source) {
313 if (target == predecessors[target])
break;
318 auto cost = distances[target] - distances[predecessors[target]];
319 auto vertex_id = graph.graph[predecessors[target]].id;
320 auto edge_id = normal?
321 graph.get_edge_id(predecessors[target], target, cost)
322 : graph.get_edge_id(target, predecessors[target], cost);
328 distances[target] - cost});
329 target = predecessors[target];
337 #endif // INCLUDE_CPP_COMMON_BASEPATH_SSEC_HPP_
Path_t & operator[](size_t i)
std::deque< Path_t > path
Path inf_cost_on_restriction(const pgrouting::trsp::Rule &rule)
void push_back(Path_t data)
Path_t set_data(int64_t d_from, int64_t d_to, int64_t d_vertex, int64_t d_edge, double d_cost, double d_tot_cost)
void empty_path(unsigned int d_vertex)
size_t countInfinityCost() const
const Path_t & operator[](size_t i) const
Path getSubpath(unsigned int j) const
void push_front(Path_t data)
std::deque< Path_t >::iterator pthIt
void appendPath(const Path &o_path)
Path(const G &graph, const V v_source, const V v_target, const std::vector< V > &predecessors, const std::vector< double > &distances, bool only_cost, bool normal=true)
void start_id(int64_t value)
const Path_t & back() const
void end_id(int64_t value)
Path(const G &graph, const Path &original, bool only_cost)
bool has_restriction(const pgrouting::trsp::Rule &rule) const
void sort_by_node_agg_cost()
Sorts a path by node, aggcost ascending.
Path(G &graph, V v_source, double distance, const std::vector< V > &predecessors, const std::vector< double > &distances)
void get_pg_dd_path(General_path_element_t **ret_path, size_t &sequence) const
void get_pg_ksp_path(General_path_element_t **ret_path, size_t &sequence, int routeId) const
std::deque< Path_t >::const_iterator ConstpthIt
Path(int64_t s_id, int64_t e_id)
void complete_path(const G &graph, const V v_source, const V v_target, const std::vector< V > &predecessors, const std::vector< double > &distances, bool normal)
constructs a path based on results
const Path_t & front() const
void generate_postgres_data(General_path_element_t **postgres_data, size_t &sequence) const
graph::Pgr_base_graph< BG, XY_vertex, Basic_edge > G
friend size_t collapse_paths(General_path_element_t **ret_path, const std::deque< Path > &paths)
friend size_t count_tuples(const std::deque< Path > &paths)
counts the tuples to be returned
friend void equi_cost(std::deque< Path > &paths)
discards common vertices with greater agg_cost
friend std::ostream & operator<<(std::ostream &log, const Path &p)
void get_pg_turn_restricted_path(General_path_element_t **ret_path, size_t &sequence, int routeId) const
boost::graph_traits< BG >::vertex_descriptor V
void append(const Path &other)
Path: 2 -> 9 seq node edge cost agg_cost 0 2 4 1 0 1 5 8 1 1 2 6 9 1 2 3 9 -1 0 3 Path: 9 -> 3 seq no...
Path(G &graph, int64_t source, double distance, const std::vector< V > &predecessors, const std::vector< double > &distances)
Path & renumber_vertices(int64_t value)
bool isEqual(const Path &subpath) const
void recalculate_agg_cost()
ConstpthIt find_restriction(const pgrouting::trsp::Rule &rule) const
get the iterator of the path where the (restriction) rule starts