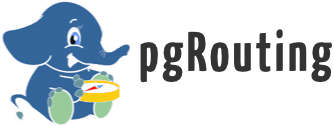 |
PGROUTING
3.2
|
Go to the documentation of this file.
50 for (
const auto e : path) {
51 (*postgres_data)[sequence] = {
62 route_cost += path[i].cost;
73 std::deque< Path > &paths) {
77 double route_cost = 0;
78 for (
auto &p : paths) {
79 p.recalculate_agg_cost();
81 for (
const Path &path : paths) {
83 get_path(route_id, path_id, path, ret_path, route_cost, sequence);
92 int64_t* via_vidsArr,
size_t size_via_vidsArr,
96 Routes_t** return_tuples,
size_t* return_count,
101 std::ostringstream log;
102 std::ostringstream err;
103 std::ostringstream notice;
115 std::deque< Path >paths;
116 log <<
"\nInserting vertices into a c++ vector structure";
117 std::vector< int64_t > via_vertices(
118 via_vidsArr, via_vidsArr + size_via_vidsArr);
121 log <<
"\nWorking with directed Graph";
132 log <<
"\nWorking with Undirected Graph";
147 (*return_tuples) = NULL;
151 *log_msg =
pgr_msg(notice.str().c_str());
156 (*return_tuples) =
pgr_alloc(count, (*return_tuples));
157 log <<
"\nConverting a set of paths into the tuples";
158 (*return_count) = (
get_route(return_tuples, paths));
159 (*return_tuples)[count - 1].edge = -2;
161 *log_msg = log.str().empty()?
164 *notice_msg = notice.str().empty()?
168 (*return_tuples) =
pgr_free(*return_tuples);
170 err << except.
what();
171 *err_msg =
pgr_msg(err.str().c_str());
172 *log_msg =
pgr_msg(log.str().c_str());
173 }
catch (std::exception &except) {
174 (*return_tuples) =
pgr_free(*return_tuples);
176 err << except.what();
177 *err_msg =
pgr_msg(err.str().c_str());
178 *log_msg =
pgr_msg(log.str().c_str());
180 (*return_tuples) =
pgr_free(*return_tuples);
182 err <<
"Caught unknown exception!";
183 *err_msg =
pgr_msg(err.str().c_str());
184 *log_msg =
pgr_msg(log.str().c_str());
T * pgr_alloc(std::size_t size, T *ptr)
allocates memory
size_t count_tuples(const std::deque< Path > &paths)
char * pgr_msg(const std::string &msg)
virtual const char * what() const
#define pgassert(expr)
Uses the standard assert syntax.
void insert_edges(const T *edges, size_t count)
Inserts count edges of type T into the graph.
static size_t get_route(Routes_t **ret_path, std::deque< Path > &paths)
An assert functionality that uses C++ throw().
static void get_path(int route_id, int path_id, const Path &path, Routes_t **postgres_data, double &route_cost, size_t &sequence)
void do_pgr_dijkstraVia(pgr_edge_t *data_edges, size_t total_edges, int64_t *via_vidsArr, size_t size_via_vidsArr, bool directed, bool strict, bool U_turn_on_edge, Routes_t **return_tuples, size_t *return_count, char **log_msg, char **notice_msg, char **err_msg)
void pgr_dijkstraVia(G &graph, const std::vector< int64_t > &via_vertices, std::deque< Path > &paths, bool strict, bool U_turn_on_edge, std::ostringstream &log)
Extends std::exception and is the exception that we throw if an assert fails.