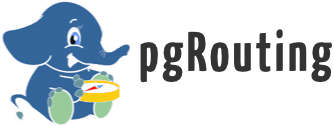 |
PGROUTING
3.2
|
Go to the documentation of this file.
26 #ifndef INCLUDE_TSP_EUCLIDEANDMATRIX_H_
27 #define INCLUDE_TSP_EUCLIDEANDMATRIX_H_
44 const std::vector< Coordinate_t > &data_coordinates);
57 void set(
size_t i,
size_t j,
double dist) {
66 bool has_id(int64_t
id)
const;
80 int64_t
get_id(
size_t idx)
const;
101 const std::vector<double>
get_row(
size_t idx)
const;
104 double distance(
size_t i,
size_t j)
const;
124 #endif // INCLUDE_TSP_EUCLIDEANDMATRIX_H_
std::vector< int64_t > ids
double comparable_distance(size_t i, size_t j) const
bool is_symmetric() const
const std::vector< double > get_row(size_t idx) const
returns a row of distances
double distance(size_t i, size_t j) const
friend std::ostream & operator<<(std::ostream &log, const EuclideanDmatrix &matrix)
size_t get_index(int64_t id) const
original id -> idx
int64_t get_id(size_t idx) const
idx -> original id
std::vector< Coordinate_t > coordinates
bool obeys_triangle_inequality() const
void set(size_t i, size_t j, double dist)
sets a special value for the distance(i,j)
EuclideanDmatrix()=default
bool has_no_infinity() const
bool has_id(int64_t id) const
original id -> true
double tourCost(const Tour &tour) const
tour evaluation
Book keeping class for swapping orders between vehicles.