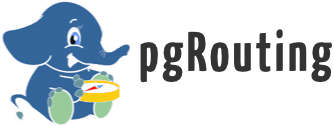 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_FLEET_H_
29 #define INCLUDE_VRP_FLEET_H_
44 class Pgr_pickDeliver;
51 typedef std::vector<Vehicle_pickDeliver>::iterator
iterator;
60 Fleet(
const std::vector<Vehicle_t> &vehicles,
double factor);
97 std::vector<Vehicle_t> vehicles,
118 #endif // INCLUDE_VRP_FLEET_H_
Identifiers< size_t > m_used
Fleet & operator=(const Fleet &fleet)
static Pgr_pickDeliver * problem
The problem.
bool is_order_ok(const Order &order) const
Given an order, Cycle trhugh all the trucks to verify if the order can be served by at least one truc...
Identifiers< size_t > m_un_used
void add_vehicle(Vehicle_t, double factor, const Vehicle_node &, const Vehicle_node &)
Extend Tw_node to evaluate the vehicle at node level.
static Pgr_messages & msg()
the problem message
std::vector< Vehicle_pickDeliver >::iterator iterator
std::vector< Vehicle_pickDeliver > m_trucks
Vehicle_pickDeliver get_truck()
friend std::ostream & operator<<(std::ostream &log, const Fleet &v)
void set_compatibles(const PD_Orders &orders)
Vehicle_pickDeliver & operator[](size_t i)
Book keeping class for swapping orders between vehicles.
bool build_fleet(std::vector< Vehicle_t > vehicles, double factor)
build the fleet