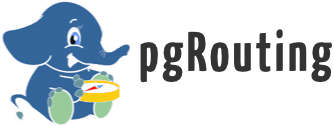 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_VEHICLE_NODE_H_
29 #define INCLUDE_VRP_VEHICLE_NODE_H_
179 #endif // INCLUDE_VRP_VEHICLE_NODE_H_
double m_tot_travel_time
Accumulated travel time.
double total_travel_time() const
_time spent moving between nodes by the truck
double total_service_time() const
_time spent by the truck servicing the nodes
int m_twvTot
Total count of TWV.
double departure_time() const
Truck's departure_time from this node.
bool is_end() const
is_end
int m_cvTot
Total count of CV.
double total_wait_time() const
_time spent by the truck waiting for nodes to open
Extend Tw_node to evaluate the vehicle at node level.
int cvTot() const
Truck's total times it has violated cargo limits.
double delta_time() const
delta_time = departure_time(this) - departure_time(previous)
double m_delta_time
Departure time - last nodes departure time.
static size_t pred(size_t i, size_t n)
double travel_time() const
@ {
bool has_cv(double cargoLimit) const
True when not violation.
double m_wait_time
Wait time at this node when early arrival.
void evaluate(double cargoLimit)
@ {
Extends the Node class to create a Node with time window attributes.
double m_arrival_time
Arrival time at this node.
bool is_late_arrival(double arrival_time) const
True when arrivalTime is after it closes.
double m_tot_wait_time
Accumulated wait time.
double total_time() const
Truck's travel duration up to this node.
double wait_time() const
Truck's wait_time at this node.
bool feasible(double cargoLimit) const
True doesn't have twc nor cv (including total counts)
Vehicle_node()=delete
Construct from parameters.
double arrival_time() const
Truck's arrival_time to this node.
Book keeping class for swapping orders between vehicles.
friend std::ostream & operator<<(std::ostream &log, const Vehicle_node &node)
@ {
double cargo() const
Truck's total cargo after the node was served.
bool has_twv() const
True when at this node does not violate time windows.
double m_tot_service_time