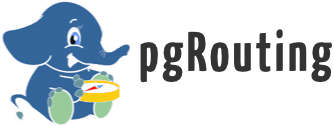 |
PGROUTING
3.2
|
Go to the documentation of this file.
51 if (
m_type ==
kStart)
return (std::numeric_limits<double>::max)();
77 case kStart:
return "START";
break;
78 case kEnd:
return "END";
break;
79 case kDump:
return "DUMP";
break;
80 case kLoad:
return "LOAD";
break;
81 case kPickup:
return "PICKUP";
break;
83 default:
return "UNKNOWN";
136 if (&other ==
this)
return true;
143 &&
id() == other.
id()
155 Dnode(id, data.pick_node_id),
157 m_opens(data.pick_open_t),
158 m_closes(data.pick_close_t),
159 m_service_time(data.pick_service_t),
160 m_demand(data.demand),
175 Dnode(id, data.start_node_id),
176 m_opens(data.start_open_t),
177 m_closes(data.start_close_t),
178 m_service_time(data.start_service_t),
The Dnode class defines a the basic operations when data is a matrix.
double travel_time_to(const Tw_node &other, double speed) const
time = distance / speed.
double distance(const Dnode &other) const
@ kDump
dump site, empties truck
NodeType m_type
The demand for the Node.
bool is_end() const
is_end
double demand() const
Returns the demand associated with this node.
std::ostream & operator<<(std::ostream &log, const Swap_info &d)
double m_opens
opening time of the node
bool is_compatible_IJ(const Tw_node &I, double speed) const
std::string type_str() const
#define pgassert(expr)
Uses the standard assert syntax.
double arrival_j_opens_i(const Tw_node &I, double speed) const
@ {
bool is_pickup() const
is_pickup
double closes() const
Returns the closing time.
bool is_delivery() const
is_delivery
@ kLoad
load site, fills the truck
An assert functionality that uses C++ throw().
int64_t m_order
order to which it belongs
double m_closes
closing time of the node
Extends the Node class to create a Node with time window attributes.
bool is_late_arrival(double arrival_time) const
True when arrivalTime is after it closes.
double opens() const
Returns the opening time.
double service_time() const
Returns the service time for this node.
bool operator==(const Tw_node &rhs) const
bool is_dump() const
is_dump
NodeType type() const
Returns the type of this node.
Book keeping class for swapping orders between vehicles.
double m_demand
The demand for the Node.