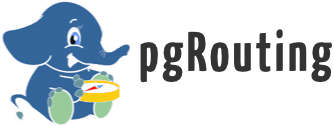 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_TW_NODE_H_
29 #define INCLUDE_VRP_TW_NODE_H_
236 #endif // INCLUDE_VRP_TW_NODE_H_
The Dnode class defines a the basic operations when data is a matrix.
double travel_time_to(const Tw_node &other, double speed) const
time = distance / speed.
@ kDump
dump site, empties truck
NodeType m_type
The demand for the Node.
bool is_end() const
is_end
friend std::ostream & operator<<(std::ostream &log, const Tw_node &node)
Print the contents of a Twnode object.
double demand() const
Returns the demand associated with this node.
double m_opens
opening time of the node
bool is_compatible_IJ(const Tw_node &I, double speed) const
std::string type_str() const
double arrival_j_opens_i(const Tw_node &I, double speed) const
@ {
bool is_early_arrival(double arrival_time) const
True when arrivalTime is before it opens.
bool is_pickup() const
is_pickup
double closes() const
Returns the closing time.
bool is_delivery() const
is_delivery
@ kLoad
load site, fills the truck
An assert functionality that uses C++ throw().
double window_length() const
Returns the length of time between the opening and closing.
int64_t m_order
order to which it belongs
double m_closes
closing time of the node
Extends the Node class to create a Node with time window attributes.
bool is_late_arrival(double arrival_time) const
True when arrivalTime is after it closes.
double opens() const
Returns the opening time.
double service_time() const
Returns the service time for this node.
void demand(double value)
bool operator==(const Tw_node &rhs) const
bool is_dump() const
is_dump
NodeType type() const
Returns the type of this node.
Book keeping class for swapping orders between vehicles.
double m_demand
The demand for the Node.