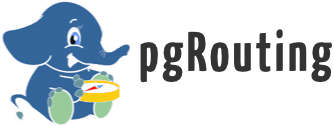 |
PGROUTING
3.2
|
Go to the documentation of this file.
102 log << static_cast<const Tw_node&>(v)
104 <<
", twvTot = " << v.
twvTot()
105 <<
", cvTot = " << v.
cvTot()
106 <<
", cargo = " << v.
cargo()
133 m_tot_travel_time(0),
134 m_tot_service_time(0) {
double m_tot_travel_time
Accumulated travel time.
int m_twvTot
Total count of TWV.
double departure_time() const
Truck's departure_time from this node.
int m_cvTot
Total count of CV.
Extend Tw_node to evaluate the vehicle at node level.
int cvTot() const
Truck's total times it has violated cargo limits.
double demand() const
Returns the demand associated with this node.
std::ostream & operator<<(std::ostream &log, const Swap_info &d)
double m_delta_time
Departure time - last nodes departure time.
bool is_early_arrival(double arrival_time) const
True when arrivalTime is before it opens.
static size_t pred(size_t i, size_t n)
double travel_time() const
@ {
bool has_cv(double cargoLimit) const
True when not violation.
double m_wait_time
Wait time at this node when early arrival.
void evaluate(double cargoLimit)
@ {
Extends the Node class to create a Node with time window attributes.
double m_arrival_time
Arrival time at this node.
double m_tot_wait_time
Accumulated wait time.
double opens() const
Returns the opening time.
double service_time() const
Returns the service time for this node.
double wait_time() const
Truck's wait_time at this node.
Vehicle_node()=delete
Construct from parameters.
double arrival_time() const
Truck's arrival_time to this node.
bool is_dump() const
is_dump
Book keeping class for swapping orders between vehicles.
double cargo() const
Truck's total cargo after the node was served.
bool has_twv() const
True when at this node does not violate time windows.
double m_tot_service_time