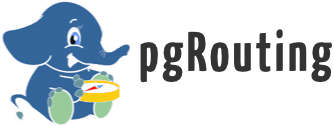 |
PGROUTING
3.2
|
Go to the documentation of this file.
32 #ifndef INCLUDE_CPP_COMMON_PGR_BIDIRECTIONAL_HPP_
33 #define INCLUDE_CPP_COMMON_PGR_BIDIRECTIONAL_HPP_
37 #include <boost/config.hpp>
39 #include <boost/graph/dijkstra_shortest_paths.hpp>
40 #include <boost/graph/adjacency_list.hpp>
58 namespace bidirectional {
61 template <
typename G >
68 typedef typename std::priority_queue<
70 std::vector<Cost_Vertex_pair>,
77 INF((std::numeric_limits<double>::max)()),
79 m_log <<
"constructor\n";
104 m_log <<
"initializing\n";
123 m_log <<
"bidir_astar\n";
140 if (forward_node.first ==
INF || backward_node.first ==
INF) {
147 if (backward_node.first < forward_node.first) {
152 if (
found(backward_node.second)) {
160 if (
found(forward_node.second)) {
184 m_log << forward_path;
186 m_log << backward_path;
187 forward_path.
append(backward_path);
188 auto p =
Path(
graph, forward_path, only_cost);
189 m_log << forward_path;
247 #endif // INCLUDE_CPP_COMMON_PGR_BIDIRECTIONAL_HPP_
std::vector< int64_t > forward_edge
std::vector< V > backward_predecessor
Priority_queue forward_queue
virtual void explore_backward(const Cost_Vertex_pair &node)=0
Pgr_bidirectional(G &pgraph)
V v_target
target descriptor
std::vector< bool > backward_finished
std::pair< double, V > Cost_Vertex_pair
V v_source
source descriptor
bool found(const V &node)
boost::graph_traits< BG >::edge_descriptor E
std::vector< double > forward_cost
V v_min_node
target descriptor
An assert functionality that uses C++ throw().
std::vector< bool > forward_finished
Path bidirectional(bool only_cost)
~Pgr_bidirectional()=default
std::vector< double > backward_cost
Priority_queue backward_queue
graph::Pgr_base_graph< BG, XY_vertex, Basic_edge > G
std::priority_queue< Cost_Vertex_pair, std::vector< Cost_Vertex_pair >, std::greater< Cost_Vertex_pair > > Priority_queue
boost::graph_traits< BG >::vertex_descriptor V
void append(const Path &other)
Path: 2 -> 9 seq node edge cost agg_cost 0 2 4 1 0 1 5 8 1 1 2 6 9 1 2 3 9 -1 0 3 Path: 9 -> 3 seq no...
Book keeping class for swapping orders between vehicles.
std::vector< V > forward_predecessor
std::vector< int64_t > backward_edge
virtual void explore_forward(const Cost_Vertex_pair &node)=0