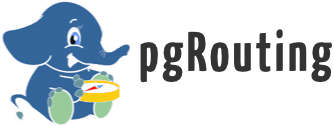 |
PGROUTING
3.2
|
Go to the documentation of this file.
25 #ifndef INCLUDE_YEN_PGR_KSP_HPP_
26 #define INCLUDE_YEN_PGR_KSP_HPP_
49 typedef std::set<Path, compPathsLess>
pSet;
70 if ((start_vertex == end_vertex) || (K == 0)) {
71 return std::deque<Path>();
77 if (!graph.has_vertex(start_vertex)
78 || !graph.has_vertex(end_vertex)) {
79 return std::deque<Path>();
88 v_source = graph.get_V(start_vertex);
135 if (
m_Heap.empty())
break;
155 if (path.
empty())
return path;
170 if (path.isEqual(rootPath)) {
171 if (path.size() > i + 1) {
172 graph.disconnect_edge(path[i].node,
181 auto spurPath = fn_dijkstra.
dijkstra(graph, spurNodeId,
m_end);
183 if (spurPath.size() > 0) {
189 graph.restore_graph();
196 for (
const auto &e : subpath)
197 graph.disconnect_vertex(e.node);
202 return std::deque<Path>();
208 paths.insert(paths.end(),
m_Heap.begin(),
m_Heap.end());
241 #endif // INCLUDE_YEN_PGR_KSP_HPP_
virtual void on_insert_to_heap(const Path) const
std::deque< Path > Yen(G &graph, int64_t start_vertex, int64_t end_vertex, size_t K, bool heap_paths)
std::deque< Path > get_results()
Path getSubpath(unsigned int j) const
pSet m_ResultSet
ordered set of shortest paths
void appendPath(const Path &o_path)
#define pgassert(expr)
Uses the standard assert syntax.
Path getFirstSolution(G &graph)
Performs the first Dijkstra of the algorithm.
std::set< Path, compPathsLess > pSet
V v_target
target descriptor
void executeYen(G &graph)
the actual algorithm
An assert functionality that uses C++ throw().
Path curr_result_path
storage for the current result
void removeVertices(G &graph, const Path &subpath)
stores in subPath the first i elements of path
virtual void on_insert_first_solution(const Path) const
graph::Pgr_base_graph< BG, XY_vertex, Basic_edge > G
V v_source
source descriptor
boost::graph_traits< BG >::vertex_descriptor V
Book keeping class for swapping orders between vehicles.
void doNextCycle(G &graph)
Performs the next cycle of the algorithm.
Path dijkstra(G &graph, int64_t start_vertex, int64_t end_vertex, bool only_cost=false)
Dijkstra 1 to 1.
void recalculate_agg_cost()