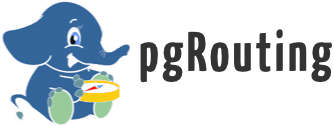 |
PGROUTING
3.2
|
Go to the documentation of this file.
28 #ifndef INCLUDE_VRP_SOLUTION_H_
29 #define INCLUDE_VRP_SOLUTION_H_
49 std::deque<Vehicle_pickDeliver>
fleet;
55 std::vector<General_vehicle_orders_t>
85 std::string
tau(
const std::string &title =
"Tau")
const;
122 #endif // INCLUDE_VRP_SOLUTION_H_
std::string tau(const std::string &title="Tau") const
static Pgr_pickDeliver * problem
this solution belongs to this problem
std::deque< Vehicle_pickDeliver > fleet
std::vector< General_vehicle_orders_t > get_postgres_result() const
Vehicle::Cost cost() const
std::string cost_str() const
Solution(const Solution &sol)
bool operator<(const Solution &s_rhs) const
double total_travel_time() const
Solution & operator=(const Solution &sol)
Initials_code get_kind() const
double total_service_time() const
friend std::ostream & operator<<(std::ostream &log, const Solution &solution)
Initials_code
Different kinds to insert an order into the vehicle.
std::tuple< int, int, size_t, double, double > Cost
Book keeping class for swapping orders between vehicles.
static Pgr_messages & msg()
The problem's message.