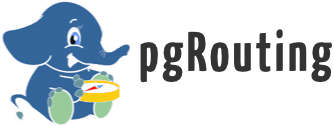 |
PGROUTING
3.2
|
#include "pgr_lineGraph.hpp"
|
typedef boost::graph_traits< G >::edge_descriptor | E |
|
typedef boost::graph_traits< G >::in_edge_iterator | EI_i |
|
typedef boost::graph_traits< G >::out_edge_iterator | EO_i |
|
typedef boost::graph_traits< G >::vertex_descriptor | V |
|
typedef boost::graph_traits< G >::vertex_iterator | V_i |
|
|
Type | boost meaning | pgRouting meaning |
G | boost::adjacency_list | Graph |
V | vertex_descriptor | Think of it as local ID of a vertex |
E | edge_descriptor | Think of it as local ID of an edge |
V_i | vertex_iterator | To cycle the vertices of the Graph |
E_i | edge_iterator | To cycle the edges of the Graph |
EO_i | out_edge_iterator | To cycle the out going edges of a vertex |
EI_i | in_edge_iterator | To cycle the in coming edges of a vertex (only in bidirectional graphs) |
|
typedef G | B_G |
|
typedef T_E | G_T_E |
|
typedef T_V | G_T_V |
|
typedef boost::graph_traits< G >::edge_iterator | E_i |
|
typedef boost::graph_traits< G >::vertices_size_type | vertices_size_type |
|
typedef boost::graph_traits< G >::edges_size_type | edges_size_type |
|
typedef boost::graph_traits< G >::degree_size_type | degree_size_type |
|
template<class G, typename T_V, typename T_E>
class pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >
Definition at line 49 of file pgr_lineGraph.hpp.
◆ B_G
template<typename G , typename T_V , typename T_E >
◆ degree_size_type
template<typename G , typename T_V , typename T_E >
template<class G , typename T_V , typename T_E >
◆ E_i
template<typename G , typename T_V , typename T_E >
◆ edges_size_type
template<typename G , typename T_V , typename T_E >
◆ EI_i
template<class G , typename T_V , typename T_E >
◆ EO_i
template<class G , typename T_V , typename T_E >
◆ G_T_E
template<typename G , typename T_V , typename T_E >
◆ G_T_V
template<typename G , typename T_V , typename T_E >
◆ id_to_V
template<typename G , typename T_V , typename T_E >
◆ IndexMap
template<typename G , typename T_V , typename T_E >
◆ LI
template<typename G , typename T_V , typename T_E >
template<class G , typename T_V , typename T_E >
◆ V_i
template<class G , typename T_V , typename T_E >
◆ vertices_size_type
template<typename G , typename T_V , typename T_E >
◆ Pgr_lineGraph() [1/2]
template<class G , typename T_V , typename T_E >
◆ Pgr_lineGraph() [2/2]
template<class G , typename T_V , typename T_E >
◆ add_one_vertex()
template<class G , typename T_V , typename T_E >
Definition at line 202 of file pgr_lineGraph.hpp.
204 auto v = add_vertex(this->
graph);
206 this->
graph[v].cp_members(vertex);
References pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::graph, pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::num_vertices(), pgassert, and pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::vertices_map.
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::insert_vertices().
◆ add_vertices()
template<typename G , typename T_V , typename T_E >
adds the vertices into the graph
PRECONDITIONS:
- The graph has not being initialized before
- There are no dupicated vertices
precondition(boost::num_vertices(
graph) == 0);
for (vertex : vertices)
POSTCONDITIONS:
postcondition(boost::num_vertices(
graph) == vertices.size());
for (vertex : vertices)
Example use:
digraph.add_vertices(vertices);
Definition at line 462 of file pgr_base_graph.hpp.
465 for (
const auto vertex : vertices) {
468 auto v = add_vertex(
graph);
470 graph[v].cp_members(vertex);
Referenced by pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges().
◆ adjacent()
template<typename G , typename T_V , typename T_E >
◆ create_edges()
template<class G , typename T_V , typename T_E >
Definition at line 169 of file pgr_lineGraph.hpp.
171 V_i vertexIt, vertexEnd;
172 EO_i e_outIt, e_outEnd;
173 EI_i e_inIt, e_inEnd;
177 for (boost::tie(vertexIt, vertexEnd) = boost::vertices(digraph.
graph);
178 vertexIt != vertexEnd; vertexIt++) {
179 auto vertex = *vertexIt;
182 for (boost::tie(e_outIt, e_outEnd) =
183 boost::out_edges(vertex, digraph.
graph);
187 for (boost::tie(e_inIt, e_inEnd) =
188 boost::in_edges(vertex, digraph.
graph);
189 e_inIt != e_inEnd; e_inIt++) {
195 (digraph.
graph[*e_inIt]).id,
196 (digraph.
graph[*e_outIt]).id);
References pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::graph, and pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::graph_add_edge().
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::Pgr_lineGraph().
◆ disconnect_edge()
template<class G , typename T_V , typename T_E >
Disconnects all edges from p_from to p_to.
- No edge is disconnected if the vertices id's do not exist in the graph
- All removed edges are stored for future reinsertion
- All parallel edges are disconnected (automatically by boost)
disconnect_edge(2,3) on an UNDIRECTED graph
disconnect_edge(2,3) on a DIRECTED graph
- Parameters
-
[in] | p_from | original vertex id of the starting point of the edge |
[in] | p_to | original vertex id of the ending point of the edge |
Definition at line 765 of file pgr_base_graph.hpp.
776 for (boost::tie(out, out_end) = out_edges(g_from,
graph);
777 out != out_end; ++out) {
778 if (
target(*out) == g_to) {
779 d_edge.id =
graph[*out].id;
782 d_edge.cost =
graph[*out].cost;
787 boost::remove_edge(g_from, g_to,
graph);
◆ disconnect_out_going_edge()
template<class G , typename T_V , typename T_E >
Disconnects the outgoing edges of a vertex.
- No edge is disconnected if it doesn't exist in the graph
- Removed edges are stored for future reinsertion
- all outgoing edges with the edge_id are removed if they exist
- Parameters
-
[in] | vertex_id | original vertex |
[in] | edge_id | original edge_id |
Definition at line 794 of file pgr_base_graph.hpp.
800 auto v_from(
get_V(vertex_id));
807 for (boost::tie(out, out_end) = out_edges(v_from,
graph);
808 out != out_end; ++out) {
809 if (
graph[*out].
id == edge_id) {
810 d_edge.id =
graph[*out].id;
813 d_edge.cost =
graph[*out].cost;
815 boost::remove_edge((*out),
graph);
◆ disconnect_vertex() [1/2]
template<class G , typename T_V , typename T_E >
Disconnects all incoming and outgoing edges from the vertex.
boost::graph doesn't recommend th to insert/remove vertices, so a vertex removal is simulated by disconnecting the vertex from the graph
- No edge is disconnected if the vertices id's do not exist in the graph
- All removed edges are stored for future reinsertion
- All parallel edges are disconnected (automatically by boost)
disconnect_vertex(2) on an UNDIRECTED graph
disconnect_vertex(2) on a DIRECTED graph
- Parameters
-
[in] | p_vertex | original vertex id of the starting point of the edge |
Definition at line 826 of file pgr_base_graph.hpp.
◆ disconnect_vertex() [2/2]
template<class G , typename T_V , typename T_E >
Definition at line 833 of file pgr_base_graph.hpp.
838 for (boost::tie(out, out_end) = out_edges(vertex,
graph);
839 out != out_end; ++out) {
840 d_edge.id =
graph[*out].id;
843 d_edge.cost =
graph[*out].cost;
850 for (boost::tie(in, in_end) = in_edges(vertex,
graph);
851 in != in_end; ++in) {
852 d_edge.id =
graph[*in].id;
855 d_edge.cost =
graph[*in].cost;
861 boost::clear_vertex(vertex,
graph);
◆ get_edge()
template<typename G , typename T_V , typename T_E >
Definition at line 671 of file pgr_base_graph.hpp.
677 V v_source, v_target;
678 double minCost = (std::numeric_limits<double>::max)();
681 for (boost::tie(out_i, out_end) = boost::out_edges(from,
graph);
682 out_i != out_end; ++out_i) {
690 if ((from == v_source) && (to == v_target)
691 && (distance ==
graph[e].cost)) {
694 if ((from == v_source) && (to == v_target)
695 && (minCost >
graph[e].cost)) {
696 minCost =
graph[e].cost;
◆ get_edge_id()
template<class G , typename T_V , typename T_E >
Definition at line 878 of file pgr_base_graph.hpp.
884 V v_source, v_target;
885 double minCost = (std::numeric_limits<double>::max)();
886 int64_t minEdge = -1;
887 for (boost::tie(out_i, out_end) = boost::out_edges(from,
graph);
888 out_i != out_end; ++out_i) {
892 if ((from == v_source) && (to == v_target)
893 && (distance ==
graph[e].cost))
895 if ((from == v_source) && (to == v_target)
896 && (minCost >
graph[e].cost)) {
897 minCost =
graph[e].cost;
898 minEdge =
graph[e].id;
901 distance = minEdge == -1? 0: minCost;
◆ get_postgres_results_directed()
template<class G , typename T_V , typename T_E >
Definition at line 91 of file pgr_lineGraph.hpp.
92 std::vector< Line_graph_rt > results;
94 typename boost::graph_traits < G >::edge_iterator edgeIt, edgeEnd;
95 std::map < std::pair<int64_t, int64_t >,
Line_graph_rt > unique;
98 for (boost::tie(edgeIt, edgeEnd) = boost::edges(this->
graph);
99 edgeIt != edgeEnd; edgeIt++) {
101 auto e_source = this->
graph[this->
source(e)].vertex_id;
102 auto e_target = this->
graph[this->
target(e)].vertex_id;
104 if (unique.find({e_target, e_source}) != unique.end()) {
105 unique[std::pair<int64_t, int64_t>(e_target,
106 e_source)].reverse_cost = 1.0;
111 if (unique.find({e_target, e_source}) != unique.end()) {
112 unique[std::pair<int64_t, int64_t>(e_target,
113 e_source)].reverse_cost = 1.0;
126 unique[std::pair<int64_t, int64_t>(e_source, e_target)] =
edge;
128 for (
const auto &
edge : unique) {
129 results.push_back(
edge.second);
References pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::graph, pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::source(), and pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::target().
◆ get_V() [1/2]
template<typename G , typename T_V , typename T_E >
get the vertex descriptor of the vertex When the vertex does not exist
- creates a new vetex
- Returns
- V: The vertex descriptor of the vertex
Definition at line 512 of file pgr_base_graph.hpp.
515 auto v = add_vertex(
graph);
516 graph[v].cp_members(vertex);
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::graph_add_edge_no_create_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::in_degree(), and pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::out_degree().
◆ get_V() [2/2]
template<typename G , typename T_V , typename T_E >
get the vertex descriptor of the vid Call has_vertex(vid) before calling this function
- Returns
- V: The vertex descriptor of the vertex
Definition at line 528 of file pgr_base_graph.hpp.
◆ graph_add_edge() [1/3]
template<class G , typename T_V , typename T_E >
template<typename T >
Definition at line 936 of file pgr_base_graph.hpp.
952 boost::tie(e, inserted) =
953 boost::add_edge(vm_s, vm_t,
graph);
961 boost::tie(e, inserted) =
962 boost::add_edge(vm_t, vm_s,
graph);
◆ graph_add_edge() [2/3]
template<class G , typename T_V , typename T_E >
template<typename T >
Definition at line 154 of file pgr_lineGraph.hpp.
163 boost::tie(e, inserted) =
164 boost::add_edge(vm_s, vm_t, this->
graph);
References pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::get_V(), pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::graph, and pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::num_edges().
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::create_edges().
◆ graph_add_edge() [3/3]
template<class G , typename T_V , typename T_E >
◆ graph_add_edge_no_create_vertex()
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ graph_add_min_edge_no_parallel()
template<class G , typename T_V , typename T_E >
template<typename T >
Definition at line 972 of file pgr_base_graph.hpp.
990 boost::tie(e1, found) =
edge(vm_s, vm_t,
graph);
997 boost::tie(e, inserted) =
998 boost::add_edge(vm_s, vm_t,
graph);
1009 boost::tie(e1, found) =
edge(vm_t, vm_s,
graph);
1016 boost::tie(e, inserted) =
1017 boost::add_edge(vm_t, vm_s,
graph);
Referenced by pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_min_edges_no_parallel().
◆ graph_add_neg_edge()
template<class G , typename T_V , typename T_E >
template<typename T >
◆ has_vertex()
template<typename G , typename T_V , typename T_E >
True when vid is in the graph.
Definition at line 534 of file pgr_base_graph.hpp.
Referenced by pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::add_vertices(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_V(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::graph_add_edge_no_create_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::in_degree(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::out_degree(), and pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::Pgr_base_graph().
◆ in_degree() [1/2]
template<typename G , typename T_V , typename T_E >
◆ in_degree() [2/2]
template<typename G , typename T_V , typename T_E >
in degree of a vertex
- when its undirected there is no "concept" of in degree
- on directed in degree of vertex is returned
Definition at line 576 of file pgr_base_graph.hpp.
578 boost::in_degree(v,
graph) :
◆ insert_edges() [1/3]
template<typename G , typename T_V , typename T_E >
template<typename T >
Inserts count edges of type pgr_edge_t into the graph The set of edges should not have an illegal vertex defined When the graph is empty calls:
- extract_vertices and throws an exception if there are illegal vertices. When developing:
- if an illegal vertex is found an exception is thrown
- That means that the set of vertices should be checked in the code that is being developed No edge is inserted when there is an error on the vertices
- Parameters
-
Definition at line 395 of file pgr_base_graph.hpp.
404 for (
const auto edge : edges) {
◆ insert_edges() [2/3]
template<typename G , typename T_V , typename T_E >
template<typename T >
Inserts count edges of type T into the graph.
Converts the edges to a std::vector<T> & calls the overloaded twin function.
- Parameters
-
Definition at line 357 of file pgr_base_graph.hpp.
Referenced by do_pgr_articulationPoints(), do_pgr_astarManyToMany(), do_pgr_bdAstar(), do_pgr_bdDijkstra(), do_pgr_bellman_ford(), do_pgr_bellman_ford_neg(), do_pgr_biconnectedComponents(), do_pgr_binaryBreadthFirstSearch(), do_pgr_bipartite(), do_pgr_boyerMyrvold(), do_pgr_breadthFirstSearch(), do_pgr_bridges(), do_pgr_combinations_dijkstra(), do_pgr_connectedComponents(), do_pgr_dagShortestPath(), do_pgr_depthFirstSearch(), do_pgr_dijkstraVia(), do_pgr_driving_many_to_dist(), do_pgr_edwardMoore(), do_pgr_floydWarshall(), do_pgr_isPlanar(), do_pgr_johnson(), do_pgr_kruskal(), do_pgr_ksp(), do_pgr_LTDTree(), do_pgr_makeConnected(), do_pgr_many_to_many_dijkstra(), do_pgr_many_withPointsDD(), do_pgr_randomSpanningTree(), do_pgr_sequentialVertexColoring(), do_pgr_stoerWagner(), do_pgr_strongComponents(), do_pgr_topologicalSort(), do_pgr_transitiveClosure(), do_pgr_turnRestrictedPath(), do_pgr_withPoints(), do_pgr_withPointsKsp(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges_neg(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_min_edges_no_parallel(), and pgrouting::alphashape::Pgr_alphaShape::Pgr_alphaShape().
◆ insert_edges() [3/3]
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_edges_neg()
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_min_edges_no_parallel() [1/2]
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_min_edges_no_parallel() [2/2]
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_negative_edges() [1/2]
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_negative_edges() [2/2]
template<typename G , typename T_V , typename T_E >
template<typename T >
◆ insert_vertices()
template<class G , typename T_V , typename T_E >
Definition at line 134 of file pgr_lineGraph.hpp.
136 auto es = boost::edges(digraph.
graph);
137 for (
auto eit = es.first; eit != es.second; ++eit) {
141 digraph[boost::source(
edge, digraph.
graph)].id,
142 digraph[boost::target(
edge, digraph.
graph)].id,
References pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::add_one_vertex(), and pgrouting::graph::Pgr_base_graph< G, T_V, T_E >::graph.
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::Pgr_lineGraph().
◆ is_directed()
template<typename G , typename T_V , typename T_E >
◆ is_source()
template<typename G , typename T_V , typename T_E >
◆ is_target()
template<typename G , typename T_V , typename T_E >
◆ is_undirected()
template<typename G , typename T_V , typename T_E >
◆ num_edges()
template<typename G , typename T_V , typename T_E >
◆ num_vertices()
template<typename G , typename T_V , typename T_E >
Definition at line 703 of file pgr_base_graph.hpp.
703 {
return boost::num_vertices(
graph);}
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::add_one_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::add_vertices(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_V(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges(), and pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::insert_vertex().
◆ operator[]() [1/4]
template<typename G , typename T_V , typename T_E >
◆ operator[]() [2/4]
template<typename G , typename T_V , typename T_E >
◆ operator[]() [3/4]
template<typename G , typename T_V , typename T_E >
◆ operator[]() [4/4]
template<typename G , typename T_V , typename T_E >
◆ out_degree() [1/2]
template<typename G , typename T_V , typename T_E >
◆ out_degree() [2/2]
template<typename G , typename T_V , typename T_E >
out degree of a vertex
regardles of undirected or directed graph
Definition at line 587 of file pgr_base_graph.hpp.
588 return boost::out_degree(v,
graph);
◆ restore_graph()
template<class G , typename T_V , typename T_E >
◆ source()
template<typename G , typename T_V , typename T_E >
Definition at line 560 of file pgr_base_graph.hpp.
560 {
return boost::source(e_idx,
graph);}
Referenced by pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::adjacent(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::apply_transformation(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_edge(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::is_source(), pgrouting::alphashape::Pgr_alphaShape::make_triangles(), and pgrouting::alphashape::Pgr_alphaShape::radius().
◆ target()
template<typename G , typename T_V , typename T_E >
Definition at line 561 of file pgr_base_graph.hpp.
561 {
return boost::target(e_idx,
graph);}
Referenced by pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::adjacent(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_edge(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::insert_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::is_target(), pgrouting::alphashape::Pgr_alphaShape::make_triangles(), and pgrouting::alphashape::Pgr_alphaShape::radius().
◆ operator<<
template<class G , typename T_V , typename T_E >
std::ostream& operator<< |
( |
std::ostream & |
log, |
|
|
const Pgr_lineGraph< G, T_V, T_E > & |
g |
|
) |
| |
|
friend |
Definition at line 69 of file pgr_lineGraph.hpp.
73 for (
auto vi = vertices(g.graph).first;
74 vi != vertices(g.graph).second; ++vi) {
75 if ((*vi) >= g.num_vertices())
break;
76 log << (*vi) <<
": " <<
" out_edges_of(" << g.graph[(*vi)] <<
"):";
77 for (boost::tie(out, out_end) = out_edges(*vi, g.graph);
78 out != out_end; ++out) {
80 << g.graph[*out].id <<
"=("
81 << g[g.source(*out)].id <<
", "
82 << g[g.target(*out)].id <<
")\t";
◆ graph
template<typename G , typename T_V , typename T_E >
The graph.
Definition at line 260 of file pgr_base_graph.hpp.
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::add_one_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::add_vertices(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::apply_transformation(), pgrouting::algorithms::articulationPoints(), pgrouting::algorithms::biconnectedComponents(), pgrouting::algorithms::bridges(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::create_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_edge(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::get_postgres_results_directed(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_V(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::graph_add_edge_no_create_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::in_degree(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::insert_vertex(), pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::insert_vertices(), pgrouting::alphashape::Pgr_alphaShape::make_triangles(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::num_edges(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::num_vertices(), pgrouting::alphashape::Pgr_alphaShape::operator()(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::operator[](), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::out_degree(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::Pgr_base_graph(), pgrouting::algorithms::pgr_connectedComponents(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::source(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::store_edge_costs(), pgrouting::algorithms::strongComponents(), and pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::target().
◆ log
template<class G , typename T_V , typename T_E >
◆ m_edges
template<class G , typename T_V , typename T_E >
◆ m_gType
template<typename G , typename T_V , typename T_E >
◆ mapIndex
template<typename G , typename T_V , typename T_E >
◆ propmapIndex
template<typename G , typename T_V , typename T_E >
◆ removed_edges
template<typename G , typename T_V , typename T_E >
◆ vertices_map
template<typename G , typename T_V , typename T_E >
id -> graph id
Definition at line 267 of file pgr_base_graph.hpp.
Referenced by pgrouting::graph::Pgr_lineGraph< G, T_V, T_E >::add_one_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::add_vertices(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::get_V(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::graph_add_edge(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::graph_add_edge_no_create_vertex(), pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::has_vertex(), pgrouting::graph::Pgr_lineGraphFull< G, T_V, T_E >::insert_vertex(), and pgrouting::graph::Pgr_base_graph< BG, XY_vertex, Basic_edge >::Pgr_base_graph().
◆ vertIndex
template<typename G , typename T_V , typename T_E >
The documentation for this class was generated from the following file:
void graph_add_edge_no_create_vertex(const T &edge)
Use this function when the vertices are already inserted in the graph.
boost::associative_property_map< IndexMap > propmapIndex
void insert_negative_edges(const T *edges, int64_t count)
degree_size_type out_degree(int64_t vertex_id) const
get the out-degree of a vertex
graphType m_gType
type (DIRECTED or UNDIRECTED)
id_to_V::const_iterator LI
bool is_source(V v_idx, E e_idx) const
boost::graph_traits< G >::in_edge_iterator EI_i
size_t check_vertices(std::vector< Basic_vertex > vertices)
#define pgassertwm(expr, msg)
Adds a message to the assertion.
bool has_vertex(int64_t vid) const
True when vid is in the graph.
#define pgassert(expr)
Uses the standard assert syntax.
boost::graph_traits< G >::edge_descriptor E
boost::graph_traits< G >::out_edge_iterator EO_i
void insert_edges(const T *edges, size_t count)
Inserts count edges of type T into the graph.
V get_V(const T_V &vertex)
get the vertex descriptor of the vertex When the vertex does not exist
bool is_target(V v_idx, E e_idx) const
void graph_add_neg_edge(const T &edge, bool normal=true)
V add_one_vertex(T_V vertex)
boost::graph_traits< G >::edge_descriptor E
size_t num_vertices() const
std::deque< T_E > removed_edges
Used for storing the removed_edges.
void add_vertices(std::vector< T_V > vertices)
adds the vertices into the graph
boost::graph_traits< G >::out_edge_iterator EO_i
bool is_undirected() const
void graph_add_min_edge_no_parallel(const T &edge)
boost::graph_traits< G >::vertex_descriptor V
void graph_add_edge(const T_E &edge)
boost::graph_traits< G >::vertex_iterator V_i
void create_edges(const pgrouting::DirectedGraph &digraph)
void graph_add_edge(const T &source, const T &target)
void disconnect_vertex(int64_t p_vertex)
Disconnects all incoming and outgoing edges from the vertex.
std::vector< Basic_vertex > extract_vertices(std::vector< Basic_vertex > vertices, const std::vector< pgr_edge_t > data_edges)
degree_size_type in_degree(int64_t vertex_id) const
boost::graph_traits< G >::in_edge_iterator EI_i
void insert_vertices(const pgrouting::DirectedGraph &digraph)
id_to_V vertices_map
id -> graph id