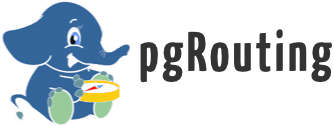 |
PGROUTING
3.2
|
Go to the documentation of this file.
48 size_t number_of_orders) :
50 m_all_orders(number_of_orders),
51 m_unassigned(number_of_orders),
83 msg().
log <<
"\nInitial_solution::do_while_foo\n";
95 msg().
log <<
"got truck:" << truck.tau() <<
"\n";
108 fleet.push_back(truck);
123 msg().
log <<
"\nInitial_solution::one_truck_all_orders\n";
135 fleet.push_back(truck);
void do_while_foo(int kind)
@ BestInsert
Insetion at the back of the truck.
@ OnePerTruck
All orders in one truck.
std::deque< Vehicle_pickDeliver > fleet
#define pgassertwm(expr, msg)
Adds a message to the assertion.
Identifiers< size_t > m_unassigned
std::ostringstream log
Stores the hint information.
Identifiers< size_t > m_assigned
@ BackTruck
Insetion at the front of the truck.
#define pgassert(expr)
Uses the standard assert syntax.
@ OneDepot
Push front order that allows more orders to be inserted at the front.
const_iterator begin() const
Identifiers< size_t > m_all_orders
An assert functionality that uses C++ throw().
Initial_solution(Initials_code kind, size_t)
@ BestBack
Best place to insert Order.
Vehicle_pickDeliver get_truck()
@ FrontTruck
One Order per truck.
@ BestFront
Push back order that allows more orders to be inserted at the back.
Initials_code
Different kinds to insert an order into the vehicle.
Book keeping class for swapping orders between vehicles.
static Pgr_messages & msg()
The problem's message.
void one_truck_all_orders()