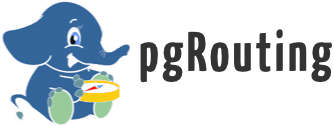 |
PGROUTING
3.2
|
Go to the documentation of this file.
56 Vehicle(idx, id, starting_site, ending_site, p_capacity, p_speed, factor),
57 cost((std::numeric_limits<double>::max)()),
58 m_orders_in_vehicle(),
86 std::ostringstream err_log;
87 err_log <<
"\n\tpickup limits (low, high) = ("
88 << pick_pos.first <<
", "
89 << pick_pos.second <<
") "
90 <<
"\n\tdeliver limits (low, high) = ("
91 << deliver_pos.first <<
", "
92 << deliver_pos.second <<
") "
93 <<
"\noriginal" <<
tau();
96 if (pick_pos.second < pick_pos.first) {
103 if (deliver_pos.second < deliver_pos.first) {
115 ++deliver_pos.second;
118 auto d_pos_backup(deliver_pos);
119 auto best_pick_pos =
m_path.size();
120 auto best_deliver_pos =
m_path.size() + 1;
122 auto min_delta_duration = (std::numeric_limits<double>::max)();
125 while (pick_pos.first <= pick_pos.second) {
127 err_log <<
"\n\tpickup cycle limits (low, high) = ("
128 << pick_pos.first <<
", "
129 << pick_pos.second <<
") ";
133 err_log <<
"\npickup inserted: " <<
tau();
136 if (deliver_pos.first <= pick_pos.first) deliver_pos.first = pick_pos.first + 1;
138 while (deliver_pos.first <= deliver_pos.second) {
143 err_log <<
"\ndelivery inserted: " <<
tau();
147 auto delta_duration =
duration() - current_duration;
148 if (delta_duration < min_delta_duration) {
150 err_log <<
"\nsuccess" <<
tau();
152 min_delta_duration = delta_duration;
153 best_pick_pos = pick_pos.first;
154 best_deliver_pos = deliver_pos.first;
160 err_log <<
"\ndelivery erased: " <<
tau();
166 err_log <<
"\npickup erased: " <<
tau();
171 deliver_pos = d_pos_backup;
173 err_log <<
"\n\trestoring deliver limits (low, high) = ("
174 << deliver_pos.first <<
", "
175 << deliver_pos.second <<
") ";
233 msg.
log <<
"unasigned" << unassigned <<
"\n";
238 while (!current_feasable.empty()) {
240 msg.
log <<
"current_feasable" << current_feasable <<
"\n";
242 auto order =
m_orders[current_feasable.front()];
248 assigned += order.idx();
249 unassigned -= order.idx();
283 assigned += order.idx();
284 unassigned -= order.idx();
286 current_feasable =
m_orders[order.idx()].subsetJ(
290 current_feasable =
m_orders[order.idx()].subsetI(
295 current_feasable -= order.idx();
324 for (
const auto &o :
orders) {
332 auto test_truck = *
this;
333 test_truck.push_back(order);
334 return test_truck.is_feasable();
352 if (deliver_pos.second < deliver_pos.first) {
363 while (deliver_pos.first <= deliver_pos.second) {
394 --deliver_pos.second;
std::deque< Vehicle_node > m_path
Identifiers< size_t > m_orders_in_vehicle
orders inserted in this vehicle
void erase(const Order &order)
const PD_Orders & orders() const
@ BestInsert
Insetion at the back of the truck.
@ OnePerTruck
All orders in one truck.
void push_back(const Order &order)
puts an order at the end of the truck
bool semiLIFO(const Order &order)
Inserts an order In semi-Lifo order.
Extend Tw_node to evaluate the vehicle at node level.
#define pgassertwm(expr, msg)
Adds a message to the assertion.
void push_front(const Order &order)
Puts an order at the end front of the truck.
std::ostringstream log
Stores the hint information.
@ BackTruck
Insetion at the front of the truck.
#define pgassert(expr)
Uses the standard assert syntax.
Identifiers< size_t > m_feasable_orders
orders that fit in the truck
@ OneDepot
Push front order that allows more orders to be inserted at the front.
void set_compatibles(const PD_Orders &orders)
Vehicle with time windows.
size_t find_best_J(Identifiers< size_t > &within_this_set) const
bool is_order_feasable(const Order &order) const
An assert functionality that uses C++ throw().
const Vehicle_node & pickup() const
The delivery node identifier.
size_t orders_size() const
@ BestBack
Best place to insert Order.
std::pair< POS, POS > drop_position_limits(const Vehicle_node node) const
const Vehicle_node & delivery() const
The delivery node identifier.
void invariant() const
Invariant The path must:
bool has(const T other) const
true ids() has element
bool insert(const Order &order)
Inserts an order.
std::pair< POS, POS > position_limits(const Vehicle_node node) const
@ FrontTruck
One Order per truck.
@ BestFront
Push back order that allows more orders to be inserted at the back.
bool has_order(const Order &order) const
void set_compatibles(double speed)
size_t find_best_I(Identifiers< size_t > &within_this_set) const
Vehicle_pickDeliver(size_t idx, int64_t id, const Vehicle_node &starting_site, const Vehicle_node &ending_site, double p_capacity, double p_speed, double factor)
Initials_code
Different kinds to insert an order into the vehicle.
void insert(POS pos, Vehicle_node node)
@ {
Book keeping class for swapping orders between vehicles.
static Pgr_messages & msg()
Access to the problem's message.
void erase(const Vehicle_node &node)
Erase node.id()
void do_while_feasable(Initials_code kind, Identifiers< size_t > &unassigned, Identifiers< size_t > &assigned)